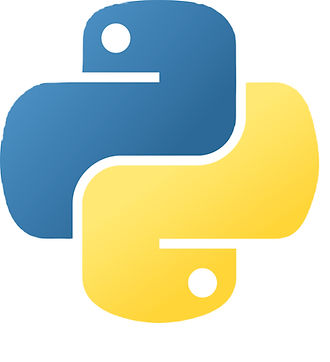
Python
Our Python programming language course offers comprehensive guidance, from fundamental concepts to advanced techniques. Receive expert support and hands-on practice to master Python effectively.
What you Learn in python
The Roadmap is divided into 29 Sections
-
Introduction and Basics of Python
-
Operators
-
Conditional Statements
-
While Loops
-
Lists
-
Strings
-
For Loop
-
Functions
-
Dictionary
-
Tuples
-
Set
-
Object-Oriented Programming
-
File Handling
-
Exception Handling
-
Regular Expression
-
Modules and Packages
-
Data Structures
-
Higher-Order Functions
-
Python Web Scrapping
-
Virtual Environment
-
Web Application Project
-
Git and GitHub
-
Deployment
-
Python Package Manager
-
Python with MongoDB Database
-
Building API
-
Statistics with NumPy
-
Data Analysis with Pandas
-
Data Visualization with Matplotlib
1 | Introduction and Basics
-
Installation
-
Python Org, Python 3
-
Variables
-
Print function
-
Input from user
-
Data Types
-
Type Conversion
-
First Program
2 | Operators
-
Arithmetic Operators
-
Relational Operators
-
Bitwise Operators
-
Logical Operators
-
Assignment Operators
-
Compound Operators
-
Membership Operators
-
Identity Operators
3 | Conditional Statements
-
If Else
-
If
-
Else
-
El If (else if)
-
If Else Ternary Expression
4 | While Loop
-
While loop logic building
-
Series based Questions
-
Break
-
Continue
-
Nested While Loops
-
Pattern-Based Questions
-
pass
-
Loop else
5 | Lists
-
List Basics
-
List Operations
-
List Comprehensions / Slicing
-
List Methods
6 | Strings
-
String Basics
-
String Literals
-
String Operations
-
String Comprehensions / Slicing
-
String Methods
7 | For Loops
-
Range function
-
For loop
-
Nested For Loops
-
Pattern-Based Questions
-
Break
-
Continue
-
Pass
-
Loop else
8 | Functions
-
Definition
-
Call
-
Function Arguments
-
Default Arguments
-
Docstrings
-
Scope
-
Special functions Lambda, Map, and Filter
-
Recursion
-
Functional Programming and Reference Functions
9 | Dictionary
-
Dictionaries Basics
-
Operations
-
Comprehensions
-
Dictionaries Methods
10 | Tuple
-
Tuples Basics
-
Tuples Comprehensions / Slicing
-
Tuple Functions
-
Tuple Methods
11 | Set
-
Sets Basics
-
Sets Operations
-
Union
-
Intersection
-
Difference and Symmetric Difference
12 | Object-Oriented Programming
-
Classes
-
Objects
-
Method Calls
-
Inheritance and Its Types
-
Overloading
-
Overriding
-
Data Hiding
-
Operator Overloading
13 | File Handling
-
File Basics
-
Opening Files
-
Reading Files
-
Writing Files
-
Editing Files
-
Working with different extensions of file
-
With Statements
14 | Exception Handling
-
Common Exceptions
-
Exception Handling
-
Try
-
Except
-
Try except else
-
Finally
-
Raising exceptions
-
Assertion
15 | Regular Expression
-
Basic RE functions
-
Patterns
-
Meta Characters
-
Character Classes
16 | Modules & Packages
-
Different types of modules
-
Inbuilt modules
-
OS
-
Sys
-
Statistics
-
Math
-
String
-
Random
-
Create your own module
-
Building Packages
-
Build your own python module and deploy it on pip
17 | Data Structures
-
Stack
-
Queue
-
Linked Lists
-
Sorting
-
Searching
-
Linear Search
-
Binary Search
18 | Higher-Order Functions
-
Function as a parameter
-
Function as a return value
-
Closures
-
Decorators
-
Map, Filter, Reduce Functions
19 | Python Web Scrapping
-
Understanding BeautifulSoup
-
Extracting Data from websites
-
Extracting Tables
-
Data in JSON format
20 | Virtual Environment
-
Virtual Environment Setup
21 | Web Application Project
-
Flask
-
Project Structure
-
Routes
-
Templates
-
Navigations
22 | Git and GitHub
-
Git - Version Control System
-
GitHub Profile building
-
Manage your work on GitHub
23 | Deployment
-
Heroku Deployment
-
Flask Integration
24 | Python Package Manager
-
What is PIP?
-
Installation
-
PIP Freeze
-
Creating Your Own Package
-
Upload it on PIP
25 | Python with MongoDB Database
-
SQL and NoSQL
-
Connecting to MongoDB URI
-
Flask application and MongoDB integration
-
CRUD Operations
-
Find
-
Delete
-
Drop
26 | Building API
-
API (Application Programming Interface)
-
Building API
-
Structure of an API
-
PUT
-
POST
-
DELETE
-
Using Postman
27 | Statistics with NumPy
-
Statistics
-
NumPy basics
-
Working with Matrix
-
Linear Algebra operations
-
Descriptive Statistics
28 | Data Analysis with Pandas
-
Data Analysis basics
-
Dataframe operations
-
Working with 2-dimensional data
-
Data Cleaning
-
Data Grouping
29 | Data Visualization with Matplotlib
-
Matplotlib Basics
-
Working with plots
-
Plot
-
Pie Chart
-
Histogram
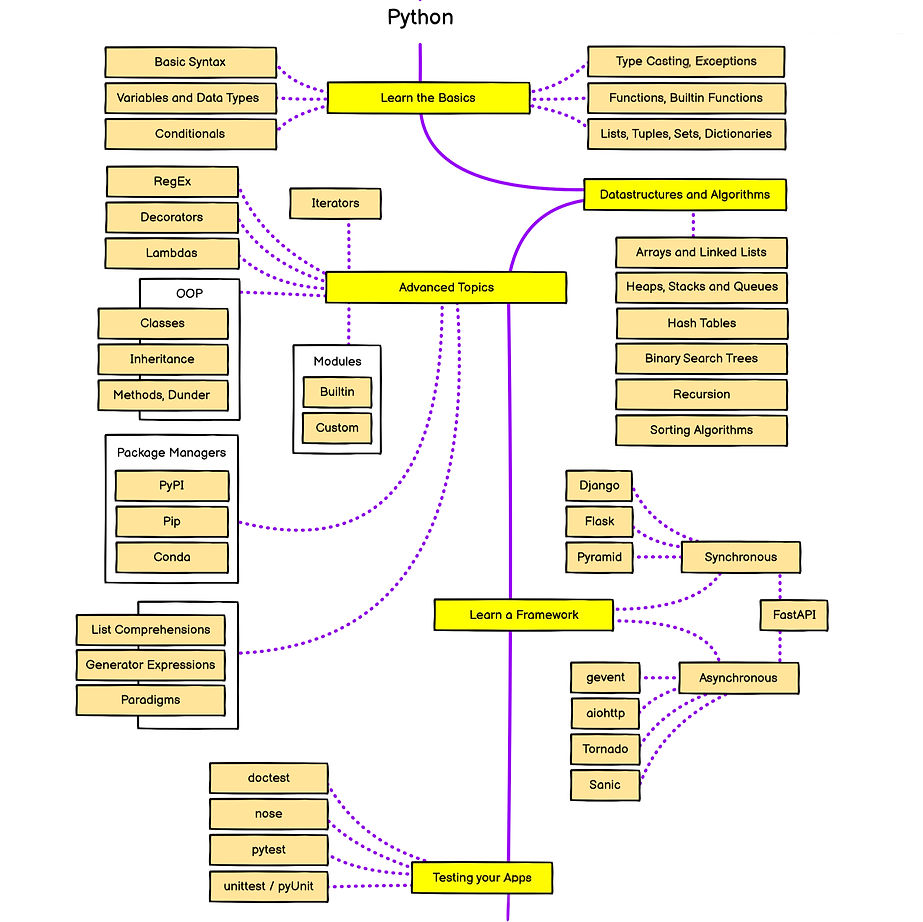